Java change textarea font
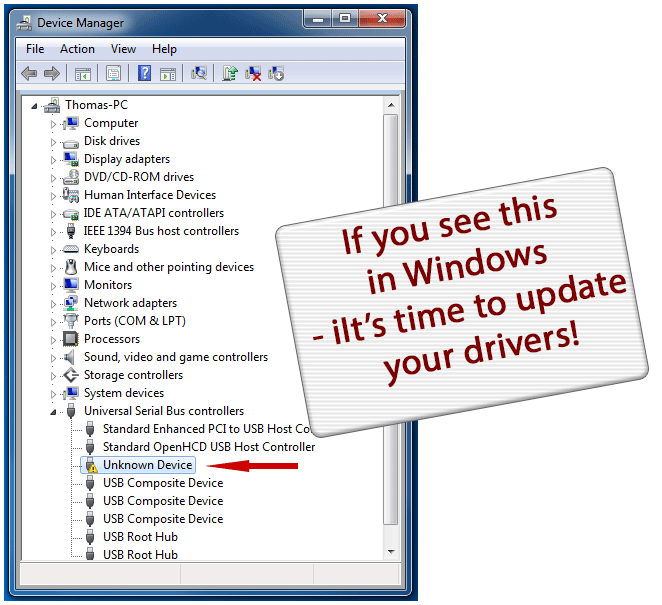
Change Font of JText Field Example This java example shows how to change font of JText Field's text using Java Swing JText Field class. import java.awt. Flow Layout; import java.awt. Font; import javax.swing. JApplet; import javax.swing. JText Field; public class JText Field Font Example extends JApplet public void init /set flow layout for the applet this.get Content Pane.set Layout(new Flow Layout /create new JText Field JText Field field = new JText Field( JText Field Change Font Example,30 To set custom font for JText Field use, void set Font( Font font) method. /create new Font Font font = new Font( Courier, Font. BOLD,12 /set font for JText Field field.set Font(font add(field.
By Alvin Alexander. Last updated: Jun 10, 2014 I'm currently writing a customized text editor in Java, and as part of that, I want to make it easy for the user to increase or decrease the font size in the text editing area (technically a JText Pane). I didn't expect this to be easy, but I've been pleasantly surprised that the following approach seems to work just fine: * Reduce the size of the font in the JText Pane editor area. public void do Smaller Font Size Action / get the current font Font f = editing Area.get Font / create a new, smaller font from the current font Font f2 = new Font(f.get Font Name f.get Style f.get Size -1 / set the new font in the editing area editing Area.set Font(f2 Java smaller font size action - discussion As you can see from that code, I do the following things: I get the current font from the JText Pane. I create the Font f2 by using that font information, but making the font size one size smaller. I set my new font on the JText Pane. This changes the font for the entire JText Pane, which is what I want. ( Although the JText Pane has many more font features, I'm just creating a simple text editor, so I'm only using one font at a time in the text editing area.) It's also worth mentioning that I have a similar method that increases the text area font size. As you can imagine, the code is identical, except I increment the font size by one instead of decrementing it. Java font size methods comes from JText Component As mentioned, I'm using this code with a JText Pane but the get Font and set Font methods both come from the JText Component class, so I expect this same approach would work with the JText Field JText Area and JEditor Pane classes as well.
Swing’s javax.swing. JText Pane class represents a significant improvement over AWT’s Text Area. It allows complex combinations of character and paragraph attributes, embedded images and components, and other features that let you build styled text editors. Unfortunately, however, while JText Pane makes complex things possible, it makes some simple things difficult. Two examples are setting the default font and color. Figure 1 shows an example of creating an AWT Text Area that uses a 20-point blue serif font. import java.awt. Text Area; import java.awt. Frame; import java.awt.event. Window Event; import java.awt.event. Window Listener; import java.awt.event. Window Adapter; import java.awt. Color; import java.awt. Font; import java.awt. Border Layout; public class Example1 extends Window Adapter public Example1 Frame f = new Frame( Text Area Example f.set Layout(new Border Layout / Make a text area, set its font and color, then add it to the frame Text Area text = new Text Area Font font = new Font( Serif, Font. ITALIC, 20 text.set Font(font text.set Foreground( Color.blue f.add(text, Border Layout. CENTER / Listen for the user to click the frame's close box f.add Window Listener(this f.set Size(400, 400 f.show public void window Closing( Window Event evt) System.exit(0 public static void main( String[] args) Example1 instance = new Example1 Figure 1. Setting text font and color on an AWT Text Area The code in Figure 1 includes a call to Text Area‘s set Font( ) and set Foreground( ) methods, which set the font and color of all of the text in the component. Figure 2 shows a (seemingly) equivalent example using Swing’s JFrame and JText Pane classes. import javax.swing. JText Pane; import javax.swing. JFrame; import java.awt.event. Window Event; import java.awt.event. Window Listener; import java.awt.event. Window Adapter; import java.awt. Color; import java.awt. Font; import.
The JText Area class provides a component that displays multiple lines of text and optionally allows the user to edit the text. If you need to obtain only one line of input from the user, you should use a text field. If you want the text area to display its text using multiple fonts or other styles, you should use an editor pane or text pane. If the displayed text has a limited length and is never edited by the user, use a label. Many of the Tutorial's examples use uneditable text areas to display program output. Here is a picture of an example called Text Demo that enables you to type text using a text field (at the top) and then appends the typed text to a text area (underneath). Click the Launch button to run Text Demo using Java™ Web Start (download JDK 7 or later). Alternatively, to compile and run the example yourself, consult the example index. You can find the entire code for this program in Text Demo.java. The following code creates and initializes the text area: text Area = new JText Area(5, 20 JScroll Pane scroll Pane = new JScroll Pane(text Area text Area.set Editable(false The two arguments to the JText Area constructor are hints as to the number of rows and columns, respectively, that the text area should display. The scroll pane that contains the text area pays attention to these hints when determining how big the scroll pane should be. Without the creation of the scroll pane, the text area would not automatically scroll. The JScroll Pane constructor shown in the preceding snippet sets up the text area for viewing in a scroll pane, and specifies that the scroll pane's scroll bars should be visible when needed. See How to Use Scroll Panes if you want further information. Text areas are editable by default. The code set Editable(false) makes the text area uneditable. It is still selectable and the user can copy data from it, but the user cannot change the text.